[AWS] Building a CI/CD Pipeline in the Cloud using Amplify, React, & GitHub
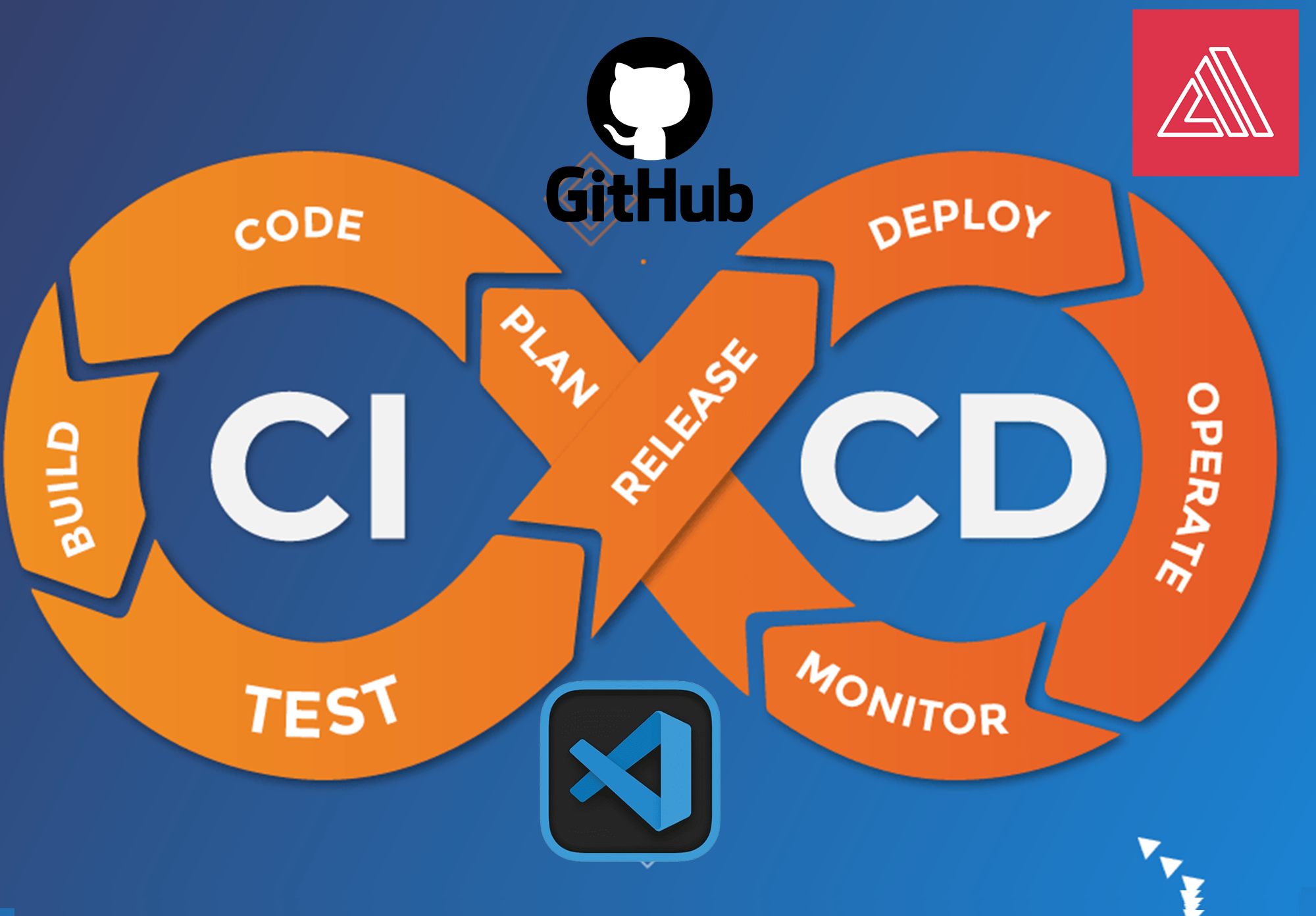
Project Overview
⭐ GitHub Repo For This Project ⭐This project focuses on developing a Continuous Integration and Continuous Deployment (CI/CD) pipeline within the AWS Cloud, featuring an AWS-based quiz game named ‘Super Tonka AWS Quiz.’ The application is built using React, integrated with Amazon Cognito, Amazon Amplify, and GitHub. The React application was developed in VS Code, with Git used for version control and to push code changes to GitHub.
Objectives:
- Establish a Robust CI/CD Pipeline: Implement a Continuous Integration and Continuous Deployment pipeline in AWS to automate the build, test, and deployment processes for the ‘Super Tonka AWS Quiz’ application.
- Develop a Scalable React Application: Create a responsive and scalable quiz application using React, ensuring a seamless user experience across various devices.
- Integrate AWS Services: Utilize AWS services such as Amazon Cognito for user authentication and Amazon Amplify for backend support, ensuring secure and efficient application functionality.
- Implement Version Control: Use Git and GitHub for version control to manage code changes, collaborate effectively, and maintain a clear history of project development.
Tools & Technologies
- VS Code (Source Repository): VS Code is simply an Integrated Development Environment (IDE) used to write computer programs of all kinds, in all types of programming languages. For this project we used React JS (JavaScript) as our primary programming language, along with some HTML and CSS code. This is our source repository where we will be developing and making changes to our source code, and it is directly connected to GitHub (Version Control).
- GitHub (Version Control): GitHub is simply a web-based platform for version control and collaboration on software development projects. It also allows us to host and manage code repositories. GitHub is the industry standard when it comes to hosting code repositories online. After we make changes to our source repository, the changes made will be ‘pushed’ to GitHub and finally these changes will be pulled from GitHub and deployed to our actual application.
- AWS Amplify: AWS Amplify is a development and deployment platform that allows developers to deploy and manage scalable mobile and web applications. After our code changes have been made and ‘pushed’ to GitHub, Amplify will automatically detect these new changes made to the code, build it and then finally deploy into the application running on Amplify.
- AWS Cognito: : A cloud-based user identity and access management service for web and mobile apps, offering scalable and secure user directories, authentication, and data storage. We will use Cognito to register and authenticate users of our quiz app
Deliverables
- Functional CI/CD Pipeline: A fully operational Continuous Integration and Continuous Deployment pipeline configured in AWS, automating the build, testing, and deployment processes for the ‘Super Tonka AWS Quiz’ application.
- React Application: A responsive and scalable quiz game built with React, hosted on a secure platform, and accessible to users via a web interface.
- Documentation (This Document): Comprehensive documentation outlining the project setup, configuration steps, user guide, and maintenance procedures, including instructions on how to modify or extend the application.
Why CI/CD?
CI/CD (Continuous Integration/Continuous Deployment) is essential for several reasons:
- Automation of Software Delivery: CI/CD automates the process of integrating code changes, running tests, and deploying applications. This reduces the risk of human error, speeds up the delivery process, and ensures that code is always in a deployable state.
- Improved Code Quality: By continuously integrating and testing code, CI/CD helps catch bugs and issues early in the development process, improving overall code quality and reducing the cost and time required for debugging.
- Faster Time-to-Market: With automated pipelines, new features, updates, and bug fixes can be deployed quickly and reliably, allowing businesses to respond to market demands and user feedback more efficiently.
Architectural Diagram
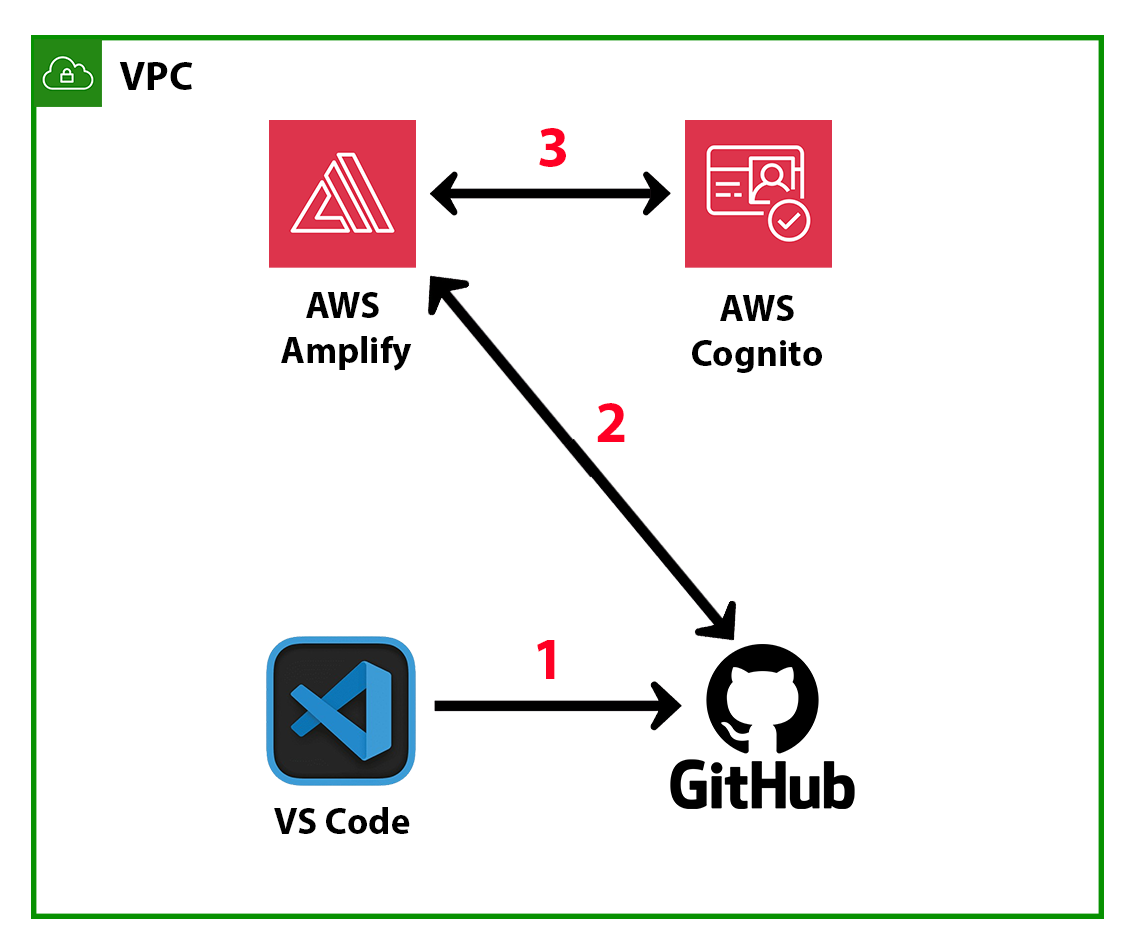
Implementation
Install & Configure the Amplify CLI (AWS)
To start off we had to make use of the command-line interface (CLI) in VS Code, which is an alternative to using the standard AWS Management Console, to create our react application. To do this we made use of a series of command-line prompts in order to configure AWS Amplify to correctly function in VS Code. We used the following script to connect to the Amazon Amplify CLI:
npm install -g @aws-amplify/cli
After, running the script we had access to the Amplify CLI and could now configure our amplify instance.
Next, we had to configure our Amplify instance and ran the following script:
amplify configure
After running the script we given some configuration options such as, logging into our own AWS account, selecting the AWS region in which to configure the Amplify instance, opening up AWS Identity Center in order to create an IAM user which will be used simply to retrieve access keys and for authentication and verification purposes.
Creating Python App in React
Now, we now need to create our actual react quiz app that will run on Amplify. We ran the following script to create a react app called ‘supertonka-react-quiz’:
npx create-react-app supertonka-react-quiz
After the react quiz app had been successfully created we then performed a few more configurations to actually initialize and get our Amplify instance ready for use. We used the script:
amplify init
Resource Creation Confirmation
That concluded the setup for Amazon Amplify using the CLI, for now. We next went over to Amazon Amplify on the actual AWS management console to confirm that our app had successfully been created. Which it was.
Adding Authentication to our app
Since our app was now ready, we proceeded to add the registration and authentication component of our app. This is where Amazon Cognito comes in. To easily integrate Cognito with our react app deployed on Amplify we simply ran the following command:
amplify add auth
This command allows us to select the registration and authentication type to be used on our app and then proceeds to implement it. This will create the ‘Sign Up’ and ‘Login’ page and logic to be used in our app . For this we chose the Email authentication method and deployed the Cognito configuration to our app with the following command:
amplify push
User Pool Creation Confirmation
To confirm that our authentication and registration system through Cognito has been successfully created we can simply take a look at our User Pools in Amazon Cognito.
Starting the app
Once we had concluded with that it was now time to actually start interacting with our react app and confirming that our login and sign-up page have successfully been created. We ran the following commands:
npm install aws-amplify @aws-amplify/ui-react
&
npm start
and proceeded to the user interface of our app which at this point was hosted locally on our machine.
Upon navigating to the user interface of our app, we are greeted with the following pages.
Adding Quiz and Answers (JavaScript)
From this we can confirm that our Cognito configuration was successful. Unfortunately, our app is blank right now and without functionality. So next we connected all our JavaScript files we had developed previously to the react app. Samples of our App.js and quizData.js files are shown below.
Once we have added our JavaScript files to the react app folder, we then saved the files and then proceeded to refresh our react app user interface. This was the outcome.
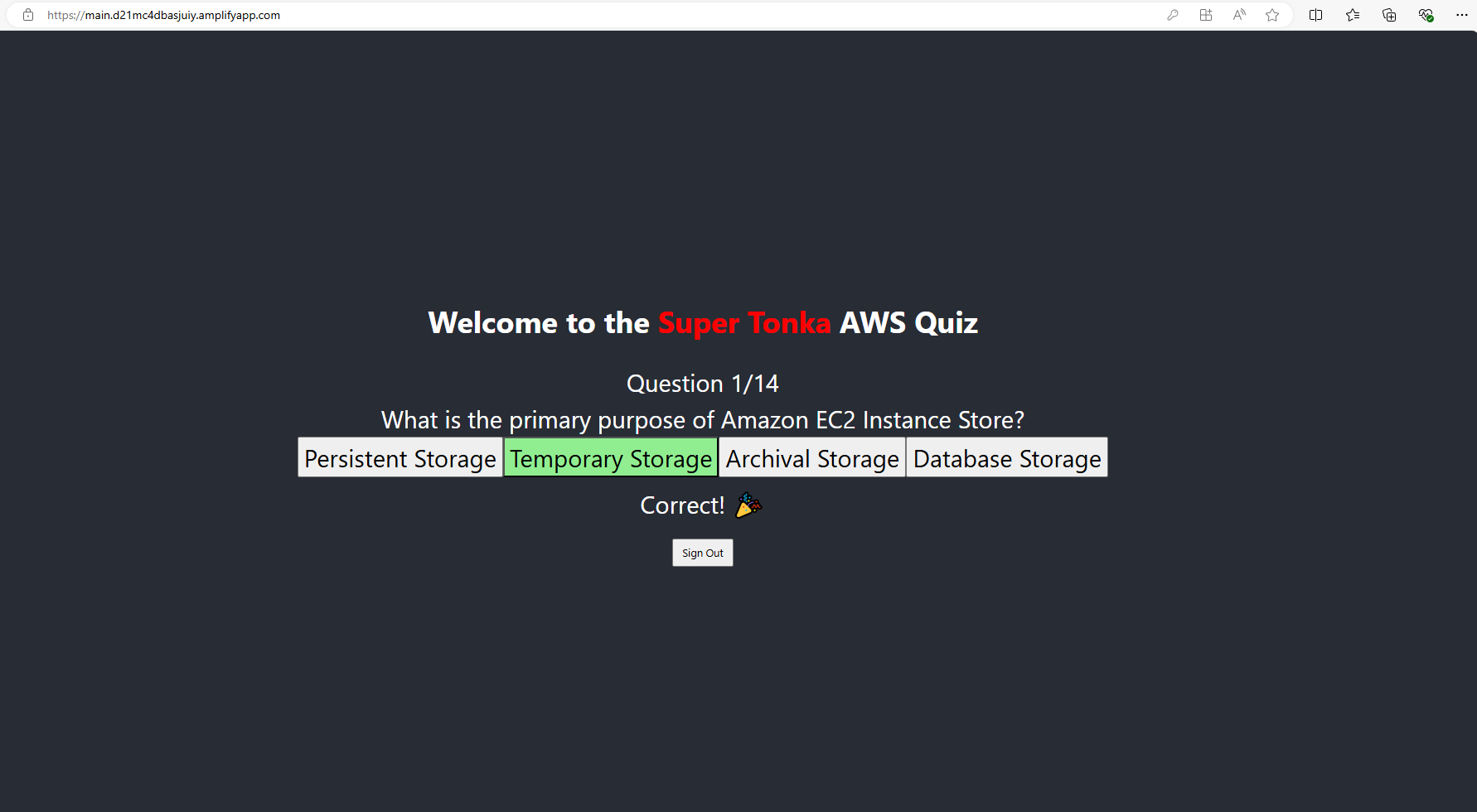
Creating GitHub Repo
Now that our react app is a success, we now need to push all our code and implementations into our version control repository (GitHub). We first logged into GitHub and created a repository called ‘AWS-Quiz-Game-with-CI/CD’.
Pushing our app to github
After we had created the repository on GitHub we went back to VS Code to ‘push’ our code into the newly created repository with the following commands:
git branch -M main
&
git push –u origin main.
At this point we had successfully pushed our code to the GitHub repository. Now the last step in the process was to connect our Amazon amplify react app with our new GitHub repository so that when we push new changes to the GitHub repository, Amazon Amplify will automatically detect changes in the GitHub repository and deploy the new changes to our react app
Configuring Amplify for CI/CD
For this we simply go back to our Amplify app in the AWS console and configure our ‘front-end environment’ to use GitHub to track changes.
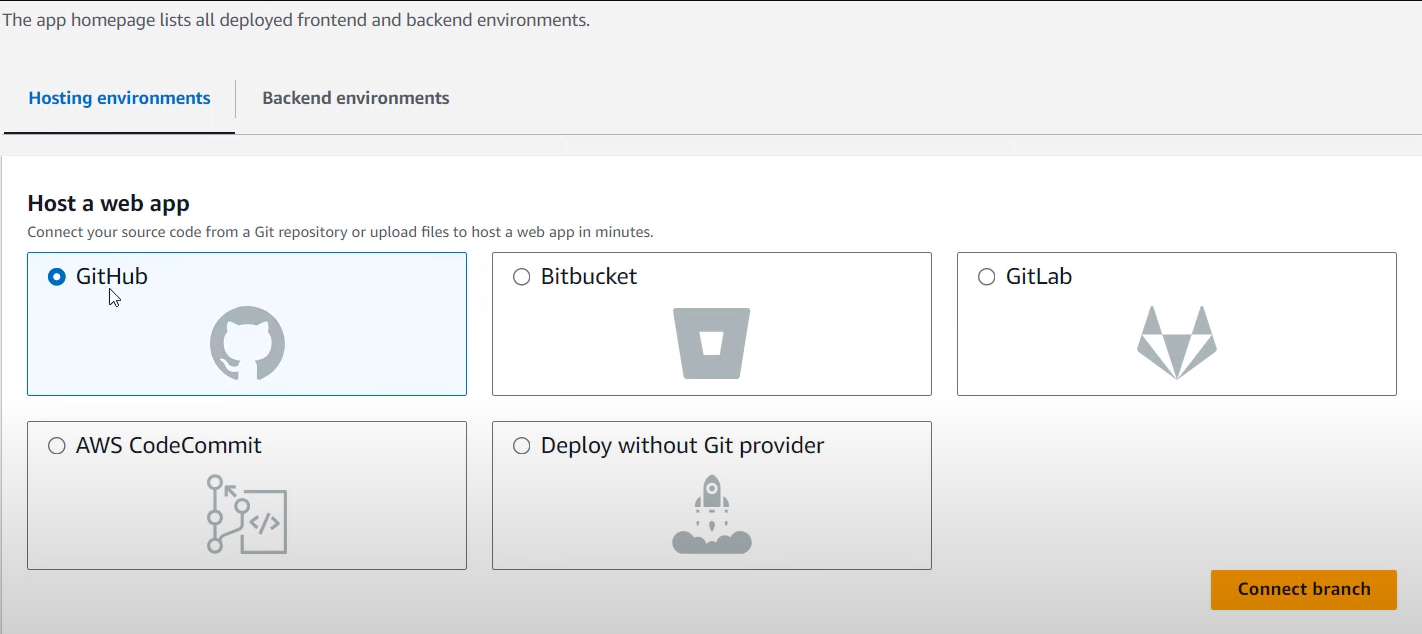
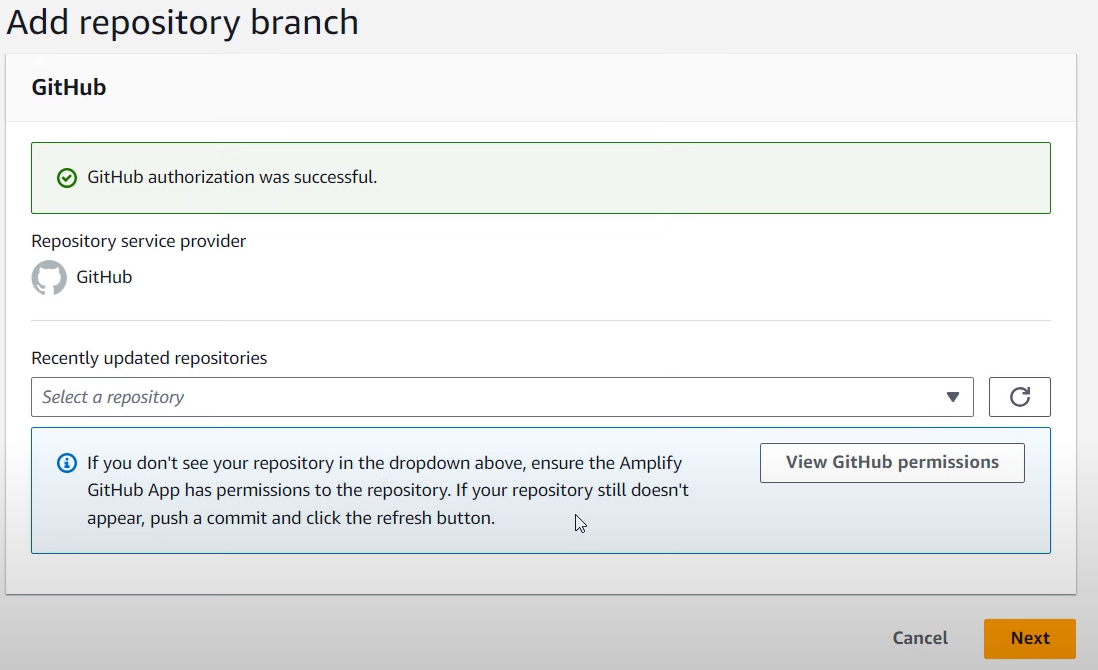
Testing CI/CD Deployment
Once Amplify was successfully connected with our GitHub repository and we had enabled it for CICD Full-Stack deployments, we can now perform a test to see what happens when we make a change to the code in our GitHub repository.
We proceeded to make a small change in our VS Code (Source Repository), we simply removed the first question from the list of quiz questions.
This was before making change to source repository…
After making changes to source repository.
As soon as some changes were made to the source repository the status of our react app changed from Deployed to Deploying, meaning that Amplify has noticed a change in the code and has begun rebuilding the solution and will deploy it as soon as it is done.
That is how CICD works, Continuous Integration (CI) happens when the solution is rebuilt once changes are made to the solution. Continuous Deployment (CD) happens when after the changes to the solution have been identified and rebuilt, the application then deploys the changes detected to the final application without any human intervention.
Challenges Faced and Solutions Implemented
Handling React Build Failures
- Challenge: Inconsistent build failures occurred due to varying Node.js versions and dependency issues between local development environments and the CI/CD pipeline..
- Solution: Standardized the Node.js version and dependencies across all environments by specifying the Node version in the package.json file and using a .nvmrc file to ensure consistency. This approach minimized discrepancies between local and pipeline builds.
Integration with GitHub
- Challenge: Maintaining smooth integration with GitHub, especially with multiple branches and pull requests, was complex and required meticulous configuration to avoid conflicts and ensure accurate deployments.
- Solution: Set up Amplify to automatically trigger builds on specific branches and configured branch-specific environments. Implemented pre-build scripts to check for merge conflicts and other issues before the deployment begins.
Conclusion & Clean Up
In this project, we successfully implemented a CI/CD pipeline using GitHub, React, and AWS Amplify. We configured GitHub for version control, integrated AWS Amplify for automated builds and deployments, and optimized React for efficient performance. By addressing key challenges and implementing effective solutions, we created a streamlined pipeline that enhances our development workflow and ensures reliable and scalable deployments.
AWS Amplify is part of Amazons free-tier and as long as your do not get 1,000,000+ requests on your amplify site, then you will be within the free-tier. For good measure it will be best to delete your deployed application and ensure your aws account does not run up and charges.