[AWS] Building a Serverless Email Marketing Application on AWS
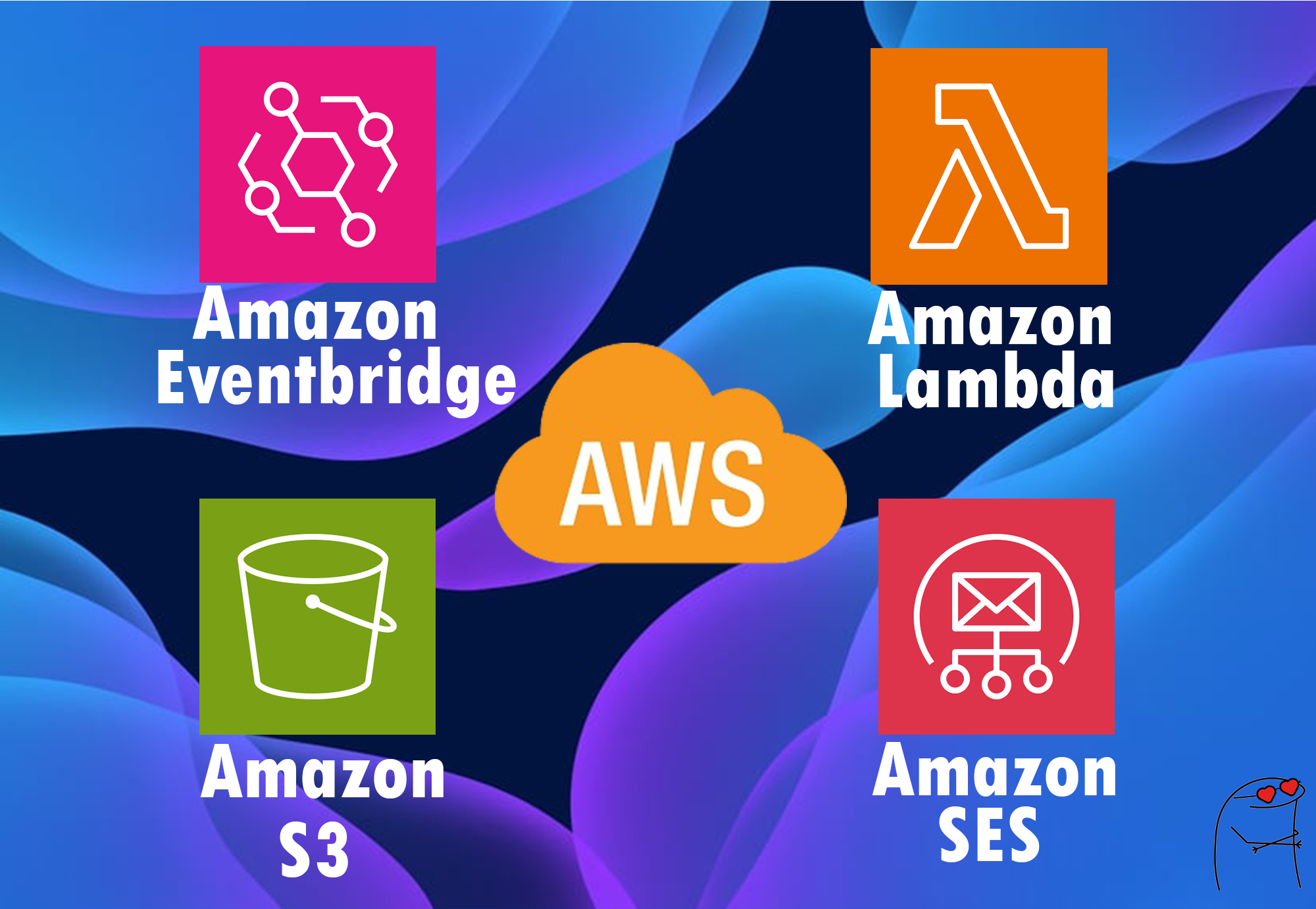
Project Overview
⭐ GitHub Repo For This Project ⭐This project involves building a Serverless Emailing Application on the AWS Cloud. The architecture used in the project is similar to real world production emailing applications hosted on the cloud. This project is serverless because there is no need to provision servers of any kind and all the actions and processes are automated and can run without any human intervention. We made use of Amazon Eventbridge to schedule weekly invocations that trigger our Lambda function to then push out mass emails through Amazon SES.
Objectives:
- Design and Implement a Serverless Architecture: Create an email marketing application using AWS services that requires no server management, ensuring scalability and high availability.
- Automate Email Campaigns: Utilize Amazon EventBridge to schedule and automate weekly email campaigns, triggered by AWS Lambda, to streamline the emailing process without human intervention.
- Leverage AWS SES for Mass Emailing: Integrate Amazon Simple Email Service (SES) to handle mass email distribution efficiently and securely within the serverless framework.
- Ensure Cost Efficiency and Scalability: Optimize the serverless infrastructure to reduce operational costs while maintaining the ability to scale according to the demand of email campaigns.
- Enhance Reliability and Security: Implement best practices for securing and monitoring the serverless application, ensuring reliable email delivery and compliance with security standards.
AWS Services Used
- Amazon S3: We had need for an object storage service that we would use to store the emailing list of all recipient’s part of our marking campaign. We also made use of S3 to store the .html file which contained our email template/styling.
- AWS Lambda: AWS Lambda is a serverless compute service which allows us to run code in response to events or invocations. Lambda will serve as the core module for this project as it will be invoked by Amazon Eventbridge and will then proceed to interact with Amazon S3 and Amazon SES.
- Amazon Eventbridge: A serverless event bus service that enables real-time event-driven applications. and custom apps to handle events and automate workflows. Amazon Eventbridge also played a key role is this project as it is the starting point to the whole operation and it is through Eventbridge that we are able to setup a weekly schedule that will invoke the Lambda function to push out our mass email.
- Amazon Simple Email Service (SES): A cloud-based email service that provides a scalable, reliable, and secure way to handle email communications, with features like content filtering and bounce/complaint handling. A self-explanatory service in which we configure all our mail servers, DNS records and configurations that allowed us to send mass emails using our own personal domains.
Deliverables
- Architectural Design Document: Detailed documentation outlining the serverless architecture, including the use of AWS services like Lambda, EventBridge, and SES.
- Deployed Serverless Email Application: A fully functional serverless email marketing application deployed on AWS, capable of automating and managing email campaigns without manual intervention.
- Automated Email Scheduling System: A system set up using Amazon EventBridge to automatically trigger email campaigns on a weekly basis.
- Lambda Functions: AWS Lambda functions configured to handle email processing and interaction with Amazon SES for email dispatch.
Why Serverless Email Marketing?
Serverless email marketing offers a cost-effective and scalable solution, enabling automated and flexible campaign management without the need for server maintenance. It allows businesses to efficiently handle varying email volumes and schedule campaigns with minimal overhead.
- Cost Efficiency: Serverless architecture eliminates the need for provisioning and maintaining servers, reducing costs as you only pay for what you use. This is ideal for email marketing, where the frequency and volume of campaigns can vary.
- Scalability: Serverless services like AWS Lambda automatically scale based on demand. This ensures that your email marketing campaigns can handle high volumes of emails without manual intervention.
- Automation and Flexibility: Serverless architectures are perfect for automating tasks, such as scheduling email campaigns and triggering emails based on specific events. This allows for more flexible and efficient email marketing strategies without the need for constant management.
Architectural Diagram
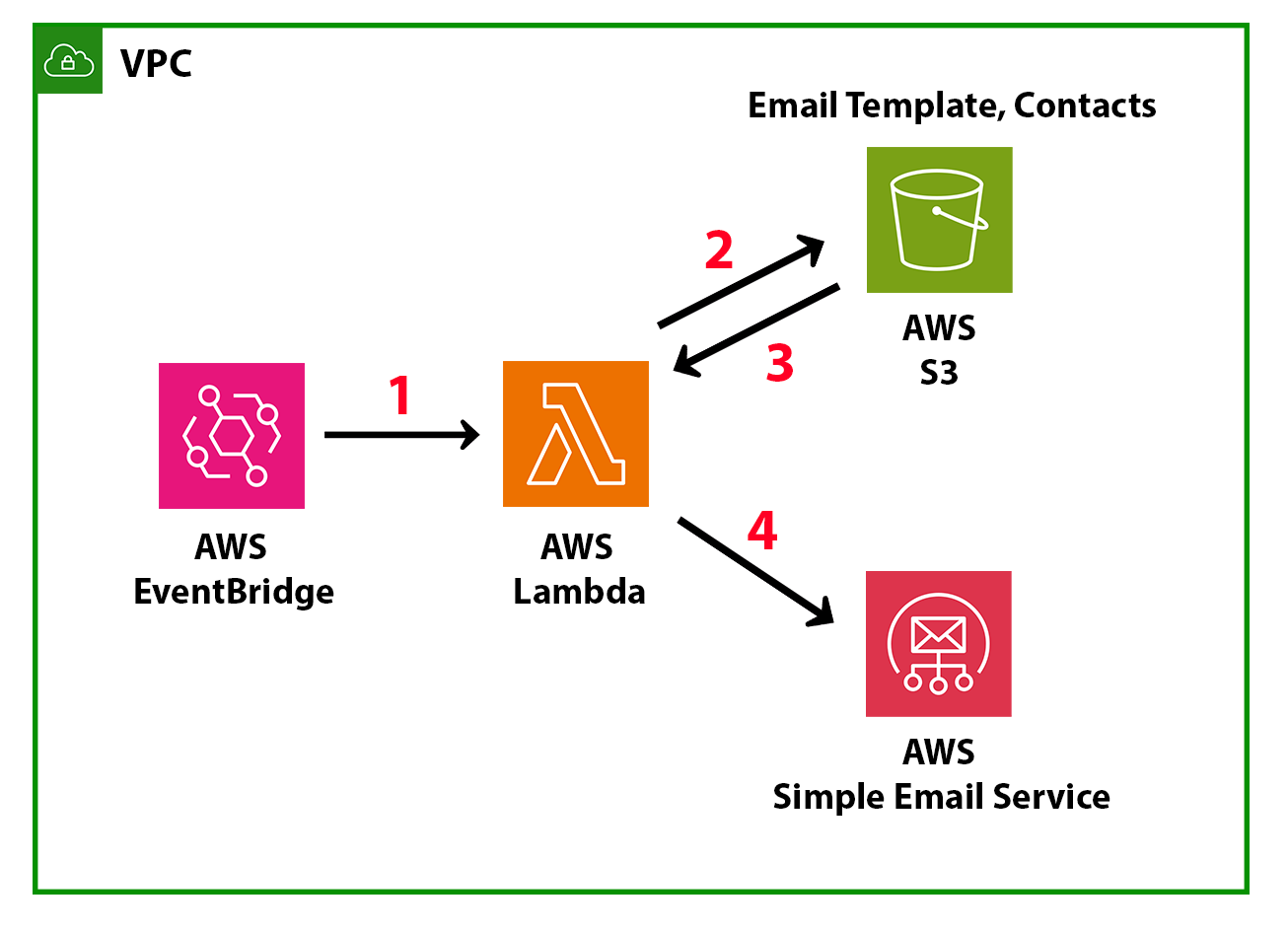
Implementation
Creating App Repo
Firstly, we had a requirement to have an object store where we will store the .html email template as well as the .csv file containing the mailing list for all the recipients of our email marketing campaign. For this we made use of a simple storage service (Amazon S3). We created a bucket called ‘serverless-email-marketing1017’, and toggled the ‘Block Public Access’ feature on to restrict unwanted access to our S3 objects.
We had our email template and email mailing list ready beforehand and proceeded to upload them to our bucket. Below is the code for email template:
all code used in this project can be found in the GitHub repository
Next, we can go over to our .csv file which was created beforehand, in which we will be placing the actual email addresses that will make up our mailing list. The 2 columns for our csv file will be ‘FirstName’ and ‘Email’.
Creating identities In Amazon SES
Next, we had to go over to Amazon SES to setup our identities, which are simply our email addresses or domains which we will use to send out emails. In the process of setting up our Amazon SES identities we initially had to enter an email that we could use to receive a verification link.
After receiving and verifying our email address the next step would be to add your sending domain, which is the primary email address from which our emails we be sent from. There is just a bit more information that needs to filled out before your SES identity is successfully setup. Once completed you should see something similar to this:
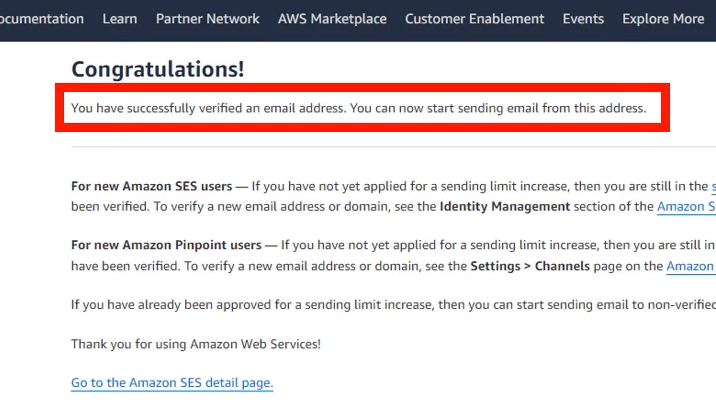
After successfully setting up the SES identities which involves editing Legacy TXT records and a lot of other DNS records we were finally able to get our email domain verified and ready to send out our email campaign. To ensure that our email domain was configured correctly we performed a test.
That concludes the setup for SES and S3 which were both targets for our Lambda function.
Creating Lambda function
Next, we then setup the Lambda function which is the core module of this project. Our lambda function was configured to retrieve the objects from our S3 bucket, merge those objects, and deliver the final product to Amazon SES. The merging process involved taking the “First Name” attribute from the mailing list and using it to replace a certain attribute within the email template in order for each email recipient to be greeted with their names like “Hi, Johnson” instead of “Hi, firstname”.
Below is the full lambda code written in python used to perform the above-mentioned actions.
If you have been following along then you know by now that before a Lambda function can retrieve or push data to other AWS services, it needs to be given permissions. Below is the IAM role (permission) written and attached to the Lambda function:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"s3:GetObject"
],
"Resource": "arn:aws:s3::: serverless-email-marketing1017/*"
},
{
"Effect": "Allow",
"Action": [
"ses:SendEmail",
"ses:SendRawEmail"
],
"Resource": "*"
}
]
}
This lambda permission set allows the lambda function to perform ‘Get’ operations on the S3 bucket named: ‘serverless-email-marketing1017’ , and allows the lambda function to perform the ‘SendEmail’ ‘SendRawEmail’ on the Amazon SES service.
Testing Lambda function
We next perform a test on lambda function to ensure everything was working as they should.
The HTTPStatus code of ‘200’ means that the test was a success.
Creating Eventbridge schedule
Next, we can move onto the final component of this architecture which is Amazon EventBridge. We will use eventbridge to invoke the function based on a scheduled action, which is similar to how mailing campaigns are sent out in real-world environments.
Once eventbridge is opened up, we are immediately greeted with the following interface in which we are to setup the schedule name and description as well as the schedule pattern.
After setting the schedule pattern up, we move on to Step 2 of creating our evenbridge schedule which entails selecting the target API which in our case will be the Lambda function called ‘send-email-to-ses’.
The next step in creating the Eventbridge schedule, we are to give our Eventbridge schedule a service role which it will use to invoke the Lambda function. We will not be creating a new service role, rather we will be allowing the service role to be automatically created as we are creating this Eventbridge schedule.
After successfully completing the process of creating the Eventbridge Schedule we get the following output on our AWS management console:
Lambda function Invokation Visualization
To sort of get a visual representation of our Lambda function actually being invoked we can navigate over to our Lambda function, scroll down and navigate over to the monitoring tab.
Once in the Monitor tab we can scroll down and take a look at some metrics relating to how many invocations of this Lambda function we have had and when these invocations were made.
We can see from these CloudWatch metrics that our Lambda function was invoked about 3 times so far and the average duration of the invocations is usually about 1.4 milliseconds. These metrics could be useful to execute performance monitoring and cost management when these Lambda functions are being invoked in a large-scale production environment.
Once the time arrives for our Eventbridge schedule to invoke our Lambda function we should receive the scheduled weekly email that should look like this:
Conclusion & Clean Up
We have successfully created a serverless email marketing application that sends out a weekly email to all users who are part of our mailing list. We were successful in creating a Lambda function that retrieves our mailing list data (emails) from an S3 bucket, after-which the Lambda function will then get invoked by an Amazon Eventbridge recurring schedule that is set to invoke the Lambda function that will then use our validated identities in Amazon SES to then forward our email template to all users who are part of our mailing list.
To conclude this project we would need to destroy all the resources we have created, so that AWS does NOT SUCK OUR POCKETS DRY!
We would have to go over to Amazon S3 and delete all the buckets created. Go over to Amazon Eventbridge and delete the recurring/one-time schedule that invokes our Lambda function . You can also go over to AWS Lambda and delete the ‘send-email-to-ses’ function and that will conclude this project finally!